Creating support tickets in Addigy scripts
You can use Addigy's go-agent binary to generate support tickets and emails from within your scripts. Here's an example of that in action.
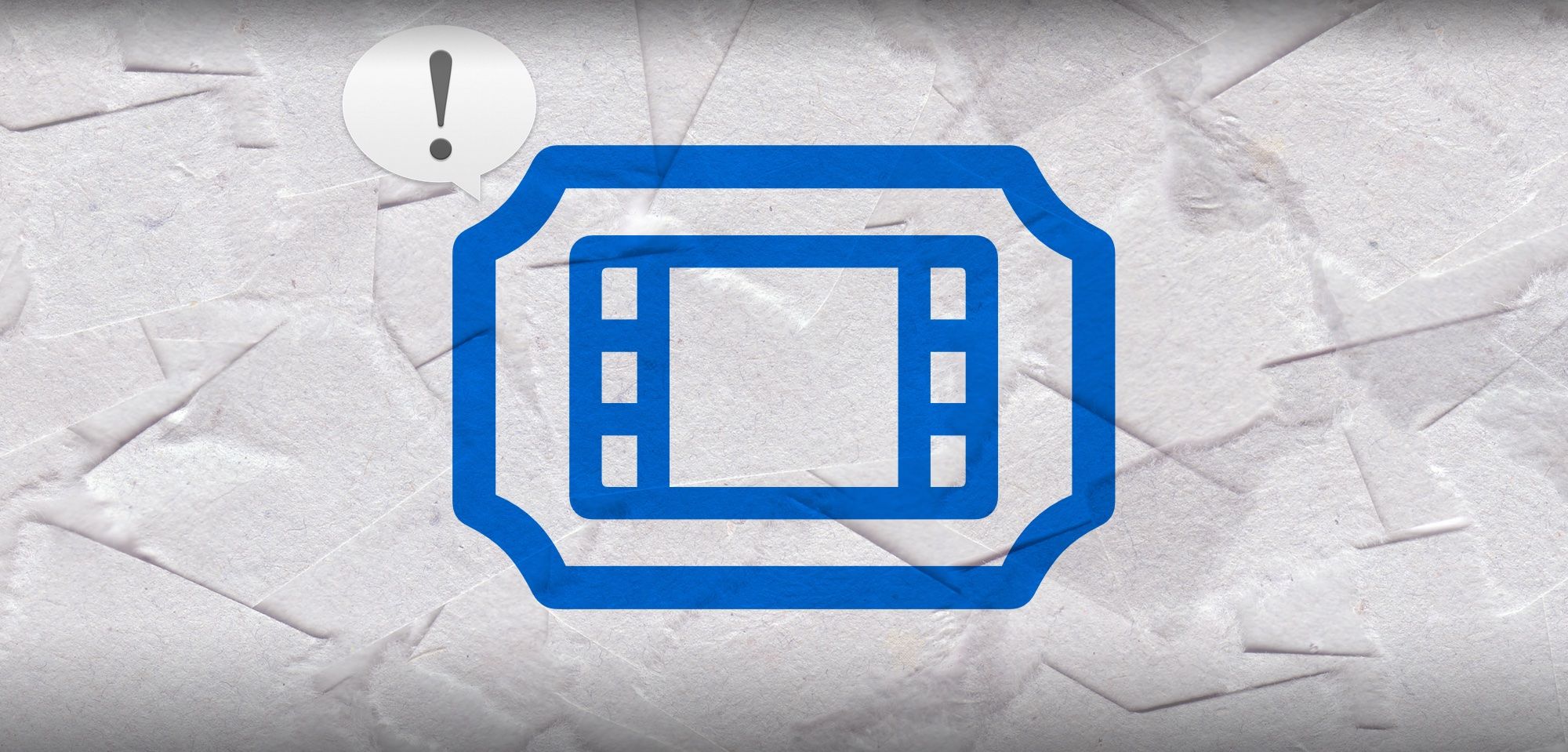
Addigy supports the ability for monitoring items (alerts) to generate support tickets or emails when triggered. This can be helpful to guide yourself or your support desk team to silently remediate issues that can't be automated away, or to reach out to an end-user to move forward. One lesser-known fact is that you can use Addigy's go-agent binary to generate support tickets, opening up this feature for use in custom software, scripts, and maintenance items.
To see this in action, here's an example of how I use go-agent to generate a support ticket with my temporary admin promotion script.
To help keep things clean, the first thing you should do is define all the variables you want to include in the ticket. This should be identifying info to help you document the event - below, we collect:
- Device hostname
- Device serial number
- Current user account name
- Current time
- Current date
# Harvest ticket information
ticketDevice=$(hostname | awk -F'.' '{print $1}')
ticketSerialNumber="$(system_profiler SPHardwareDataType | awk '/Serial/ {print $4}')"
ticketUserName="$(/usr/bin/stat -f%Su /dev/console)"
ticketTime="$(date +%r)"
ticketDate="$(date +%x)"
Aside from that, there may be other information you want to retain. In this script, since we're capturing user input on why they need admin access, we collect their response from that text box, sanitize it (remove characters that, if included, would break the upload command), set a maximum character count to prevent errors, and store it. The raw input is managed below as "response1", acted upon, and then tucked into a variable as our ticket description:
# Catch invalid user input
if [[ "$response1" == "" ]]; then
response1="User left text box empty"
fi
# Remove bad characters
# TO REMOVE: Double quotes, single quotes, backslashes
# TO KEEP: Forward slashes, colon, exclamation point, dollar sign, asterisk, parentheses, brackets, braces
response1="$(echo "$response1" | tr -d \'\"\\ )"
echo "User response with special characters removed: $response1"
# Set maximum character length to 1024
if [ ${#response1} -ge 1024 ]; then
echo "Shortening string to 1024 characters"
response1=$( echo "$response1" | cut -c 1-1024)
echo "Truncated response: $response1"
fi
ticketDescription="--- EVENT --- \n User ran Admin Promotion script with the following request: \n \n $response1"
With that done, we can finally assemble the ticket. The method I use is to wrap the command inside a variable so I can assess the output of the command after it executes.
ticketRequest="$(curl -X POST https://$(/Library/Addigy/go-agent agent realm).addigy.com/submit_ticket/ -H 'content-type: application/json' -d "{\"agentid\": \"$(/Library/Addigy/go-agent agent agentid)\", \"orgid\":\"$(/Library/Addigy/go-agent agent orgid)\", \"name\":\"$ticketUserName\", \"description\":\"\n ____________________________________________________________ \n Device name: $ticketDevice \n Serial number: $ticketSerialNumber \n Username: $ticketUserName \n Date: $ticketDate, $ticketTime \n ‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾ \n $ticketDescription \"}")"
You'll notice I've included a bit of formatting in the description field. Use "\n" to add line breaks to your output, and I'm a fan of using underscores or other similar ASCII characters to draw horizontal rules.
If you strip away all the variables we listed out, here's the remaining command. The only two things you need to provide are "$ticketUserName" and "$ticketDescription":
curl -X POST https://$(/Library/Addigy/go-agent agent realm).addigy.com/submit_ticket/ -H 'content-type: application/json' -d "{\"agentid\": \"$(/Library/Addigy/go-agent agent agentid)\", \"orgid\":\"$(/Library/Addigy/go-agent agent orgid)\", \"name\":\"$ticketUserName\", \"description\":\"$ticketDescription \"}"
What you do from here is up to you. I include a failure condition, where if the variable encasing the command equals a specific string that occurs if the upload fails, my script bails out:
# If ticket creation fails, halt - user will have to contact suppport Support Desk
if [[ "$ticketRequest" == *"Something went wrong, we are looking into this issue."* ]]; then
echo "$timestamp [RESULT] Ticket not sent, display error and exit"
else
echo "$timestamp [RESULT] Ticket created successfully, promoting."
fi
The choice is yours - just remember to collect general identifying/bookkeeping information along with the relevant info from your ticket.