Software Updates, Part 2 - Advanced States
Depending on your Macs' software update state, you can divide your fleet into groups with some advanced logic.
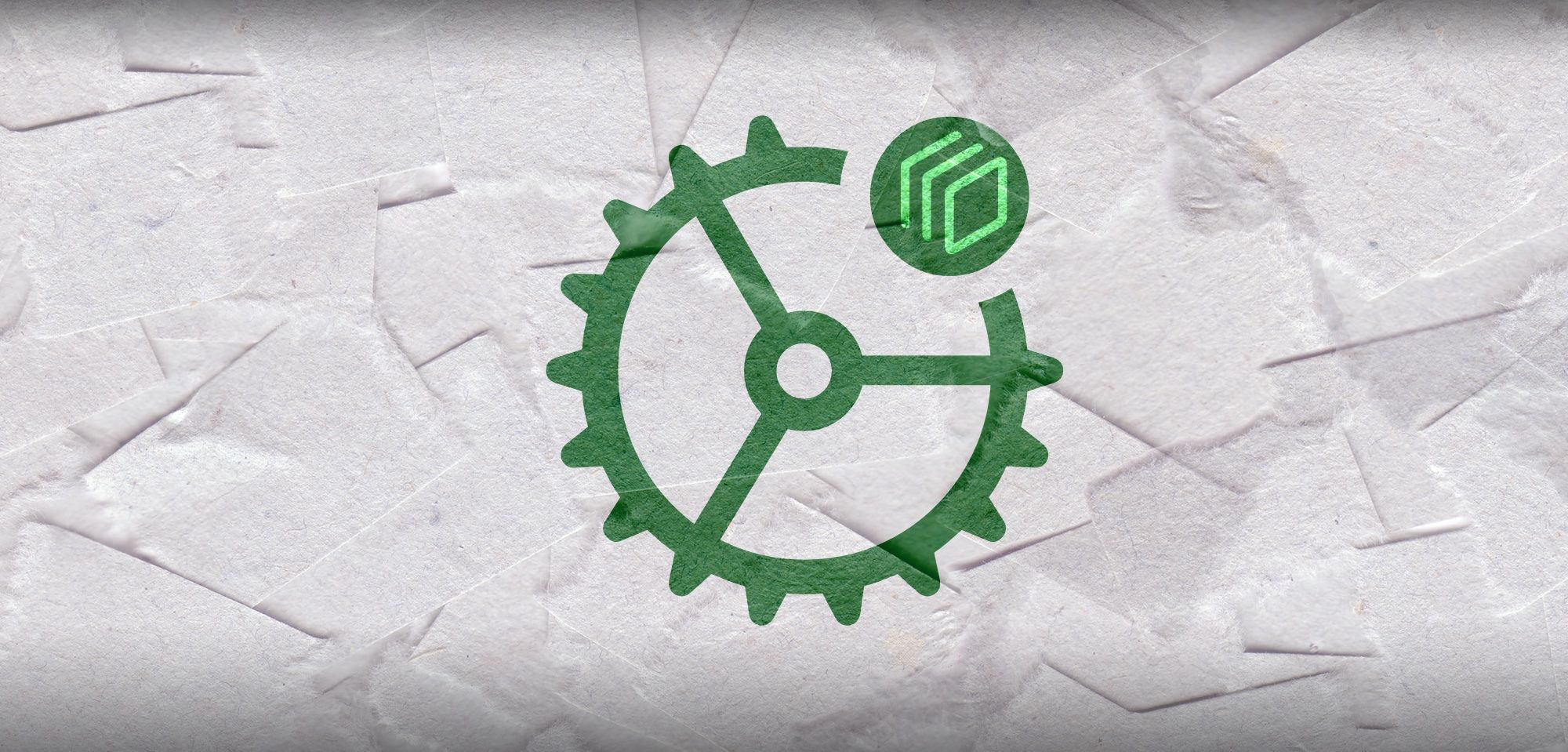
In the last post, we reviewed some ways to collect information about the software update status of your Macs. The next thing to do is assess that information, break your devices into groups, and decide what action to take.
Here's a place to begin your assessment of your devices - some environments may want to identify devices in all these states, while others will only need a few.
Beta Testers
- Enrolled in beta program = true
If you have any users in your environment who are enrolled in Apple's beta programs, their macOS versions may not align with the rest of the users in your environment. When a Mac runs the .pkg installer to enroll in the beta program, a flag file will be created, so we can test for the presence of that file:
betaFlag="/Users/Shared/.SeedEnrollment.plist"
#This file is created when enrolling in the beta program
if [[ -f "$betaFlag" ]]; then
echo "TRUE"
else
echo "FALSE"
fi
Fully Updated
- Available updates = 0
- Major macOS version = newest version
These Macs are running the newest major and minor versions of macOS - they have no available updates or upgrades for them. For most fleets, this is your desired/compliant device state.
Upgrade Recommended
- Current macOS is the newest version = false
If you try to keep all of your devices on the newest version of macOS, quickly identifying devices that aren't running that version will be important. To assess this, use this fact to check if the Mac is on the newest version of macOS that you define:
#!/bin/bash
# Upgrade Recommended assessment | macOS on newest version fact
# macOS Ventura = 13
# macOS Monterey = 12
# macOS Big Sur = 11
# macOS Catalina and earlier = 10
latestReleaseOS=13 # Update this variable each year
# Get current OS on device
currentOSMajor=$(sw_vers -productVersion | awk -F. '{print $1}')
if [[ $currentOSMajor = $latestReleaseOS ]]; then
echo "TRUE"
else
echo "FALSE"
fi
Minor Updates Complete
- Available updates = 0
- Available upgrades = any value
These Macs can be running any major version of macOS, but whatever version they have, there are no software updates available for them. In environments where you need to support multiple OS versions, this is a helpful compliance metric.
Unsupported OS
- Latest major macOS version - current major macOS version ≤ 2
Apple currently runs on an n-2 support cadence for macOS. They continue to release security updates for the newest/current major version of macOS and the two versions before that. With the release of macOS Ventura, this arithmetic is a little easier than it used to be:
#!/bin/bash
# Supported OS check
# macOS Ventura = 13
# macOS Monterey = 12
# macOS Big Sur = 11
# macOS Catalina and earlier = 10
latestReleaseOS=13 #Update this variable each year
# Get current OS on device
currentOSMajor=$(sw_vers -productVersion | awk -F. '{print $1}')
difference=$(($latestReleaseOS-$currentOSMajor))
if [[ $difference < 3 ]]; then
# Device falls into n-2 window
echo "TRUE"
else
echo "FALSE"
fi
Software Update Bugged
- Available updates = 0
- macOS minor version matches newest available = false
This is a very important status to track and will require regular updates. You'll need to define variables with the newest minor version of each major OS still being supported by Apple and compare that to the current version running on your device. If the device is not running the newest version and is claiming no updates are available, the Mac is in a bugged state. Here's a boolean you can use to see if the Mac is on the current OS - mix this with the previous fact we used to check the number of available updates to identify truly bugged Macs:
#!/bin/bash
# Running latest update available update for current supported OS
latest13="13.1"
latest12="12.6.2"
latest11="11.7.2"
# Get current OS on device
currentOS=$(sw_vers -productVersion)
currentOSMajor=$(sw_vers -productVersion | awk -F. '{print $1}')
if [[ $currentOSMajor = 13 ]]; then
# macOS Ventura (13) detected
if [[ $currentOS = $latest13 ]]; then
echo "TRUE"
else
echo "FALSE"
fi
exit 0
elif [[ $currentOSMajor = 12 ]]; then
# macOS Monterey (12) detected
if [[ $currentOS = $latest12 ]]; then
echo "TRUE"
else
echo "FALSE"
fi
exit 0
elif [[ $currentOSMajor = 11 ]]; then
# macOS Monterey (11) detected
if [[ $currentOS = $latest11 ]]; then
echo "TRUE"
else
echo "FALSE"
fi
exit 0
else
echo "FALSE"
fi
By doing this check manually and providing the expected/desired macOS version numbers, you can get accurate results regardless of what the softwareupdate
binary is saying. Again, the goal here would be to set up a smart group/flex policy that looks for the above fact to return "FALSE" and the macOS updates available fact to return "0" to identify bugged Macs. If you need to assess this in a single fact, just embed the entire above script inside an if
statement that checks for if there are any available updates - if no, assess this. If there are available updates detected, we know the Mac isn't in a bugged state.
Next steps
We now have a lot of additional information about our Macs, with more advanced scripts that can help meet our unique environments' needs. You can use this to define your support strategies - monitoring scripts to prompt users to update/upgrade, generating lists of devices to schedule support for, and setting auto-remediation scripts to try and kick devices out of bugged states.